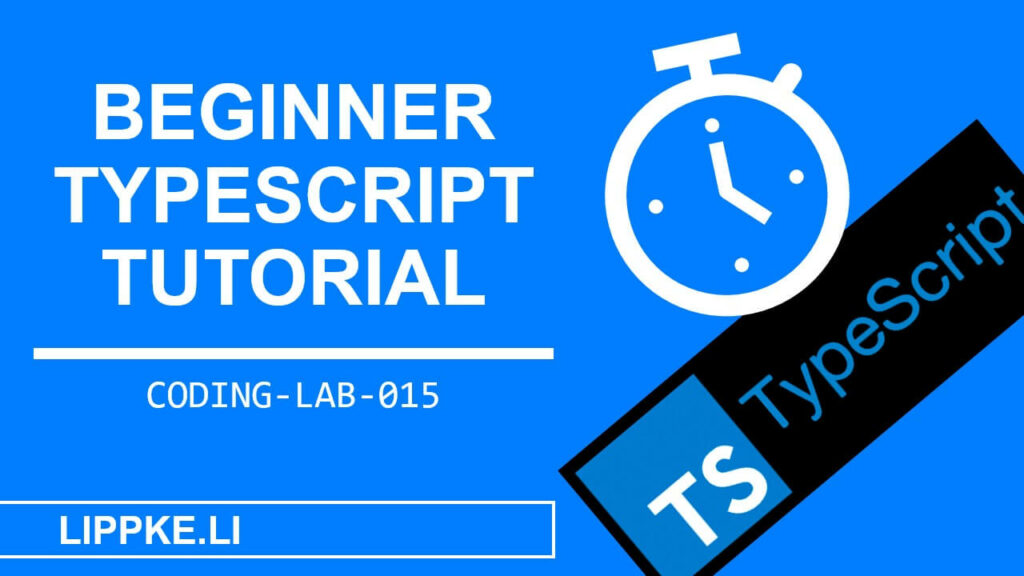
Do you need an introduction to TypeScript?
Angular, React and Ionic use this language.
What makes the language so different? How do I use TypeScript efficiently?
This guide gives you a step-by-step introduction!
Let’s get started!
Challenge: Understanding TypeScript
You can use TypeScript to create an object-orientated blog with posts, authors and comments, for example.
The trained Java developer is happy if he can programme object-oriented with TypeScript.
That would be helpful …
- Have programmed something … e.g. with JavaScript as the basis for TypeScript.
- Basics in the command line
- Admin rights to install and uninstall programmes
What you need on your computer
- Admin rights
- More than 5 GB RAM (better 12 GB – 16 GB)
- Code Editor
- optional WSL for Windows users
Example editor (Visual Studio Code)
In addition to the browser, you need a code editor of your choice.
You can use Notepad as an alternative. It is best to use an editor with syntax highlighting and IntelliSense, such as Visual Studio Code or Atom.
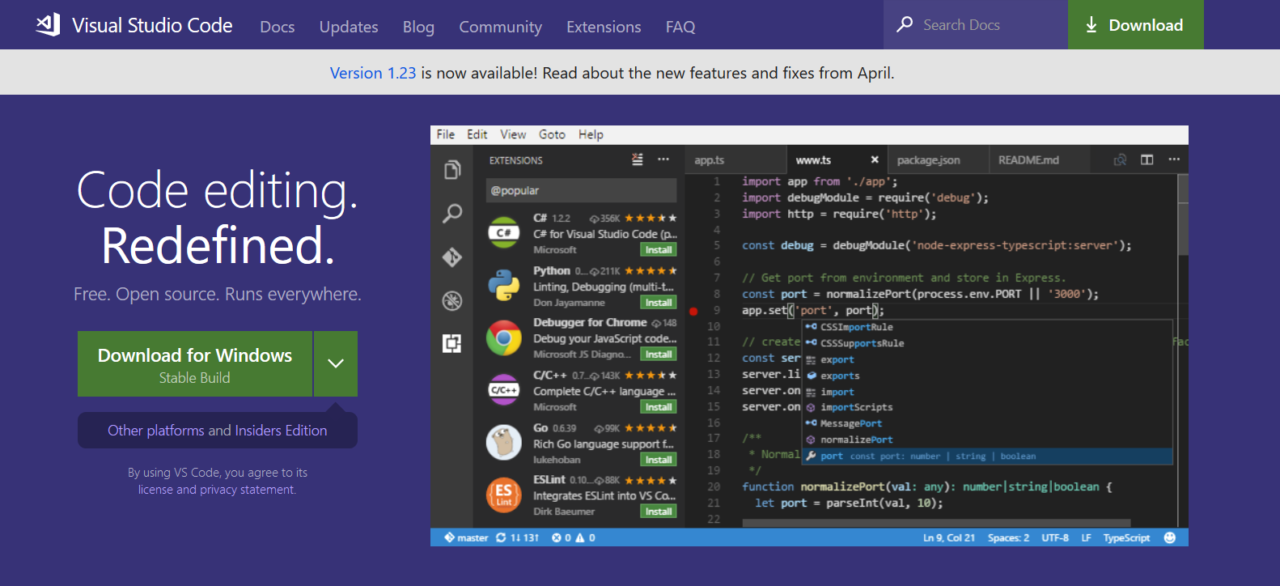
Concept: Why TypeScript is cool!
Type-safe programming helps you to keep an overview of the code.
What many beginners find annoying helps you when programming complex projects because you can keep an overview.
Microsoft designed the TypeScript programming language to bring more order to web programming.
What is typecasting anyway? TypeScript allows the programmer to use variables with a type, e.g. let date:Date; or let text:string = ""
This allows a simpler, object-orientated programming. Construct your own objects and structures.
JavaScript Next-Generation
Valid JavaScript code is valid TypeScript code!
If you know JavaScript, then you don’t need to learn much more. TypeScript extends the range of possibilities of JavaScript and offers a number of simplifications.
Angular’s strict compiler requires you to convert the type-less JavaScript code into “formulated” TypeScript code. This ensures that you always type parameters, variables and return values.
5 reasons – TypeScript is better than JavaScript
- Easier typing (optional) of variables:
Java has strict typing. The Java code assigns each variable to a type. This helps the programmer to orientate himself in the code. - Simple refactoring due to typing:
If you come up with the idea that a variable does not correspond to your naming standard, you can simply rename the variable. - ECMAScript up to 3:
The JavaScript programming language has received several updates since 1995 that extend the functional scope of the script language. The TypeScript compiler can compile the TypeScript code into JavaScript in an understandable way. - Popular and necessary:
TypeScript used in various web frameworks such as Angular and Ionic - Dependency injection:
… simplifies testing with TypeScript.
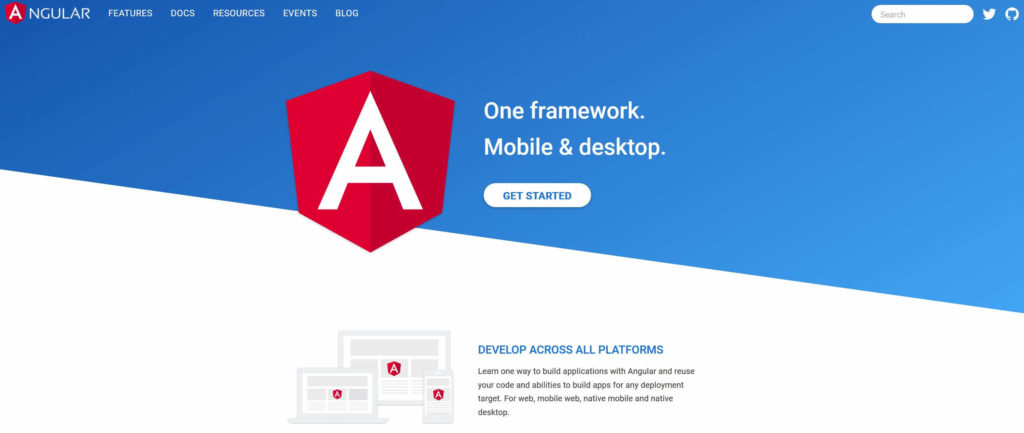
Basics: TypeScript basics
What the heck is TypeScript?
To begin with, I would like to explain a few key TypeScript terms. Classes, namespaces and modules are concepts that can help you with programming.
Understanding TypeScript classes
- Class: Classes allow you to represent the reality as an abstracted model. As a programmer, you try to transfer reality to the computer in a simplified form. A class can contain attributes, for example:
class Person{
name:string;
}
- Extends: The command extends an existing class with a super class. The subclass “inherits” all variables and functions from the super class. The
super();
function initialises the variables of the super class.class deer extend animal {
constructor( ){
super("typ3"
}
}
Managing modules – Importing and exporting namespaces
- Namespace: A namespace prevents variables with the same name from interfering with each other. A namespace can contain classes, interfaces and variables. It is possible that you can use several variables with the same name in one file. However, you should never do this because it will disrupt your orientation in the code.
namespace PersonalModule{
// new namespace without external overlap
}
- Modules: Internal modules are namespaces and external modules are normal modules. Before you import a module, you must declare an export in the source file.
- Export: The statement makes the class, interface or variable externally visible / usable.
class Person{
}
export { Person };
- Import: At the beginning of a file, the developer can use the import command to integrate modules from outside.
import { Person } from "./Person";
- Interfaces: … are combinations of variables that should always occur in a context.
Coding: Off into practice
Now it’s getting serious.
We will create your first TypeScript programme with these steps.
A little practice will help the learning process:
I call JavaScript a “beginner-friendly” programming language because the programme doesn’t crash completely straight away and remains partially functional.
Remember that JavaScript in its pure form is valid TypeScript code!
Variable types
- let variables are only accessible within the curly brackets. A call from the outside goes nowhere (undefinded).
let text:string = "my super text";
- the programmer declares const variables at the beginning of a file, which can be used globally in all functions as a constant. In Java, you differentiate between float, double, short, etc. TypeScript only recognises
number
.const PI:number = 3.141;
- var can be used as in JavaScript.
var normalVariable:number = 42;
- Typing is possible for decimal, binary, hexadecimal and octal numbers.
const hexa:number = 23a23ff;
Strings…let text:string = "This is text";
Array…let textlist:string[] = ["All",
"
are", "good"];
Iterators
- for … of
can iterate through an array and returns the VALUES("day", "night", "morning")
in the array.for(let entry of blogEntries) {
console.log(entry)
}
- for … in
can iterate through an array and returns the KEYS(0,1,2..)
in the array.for(let keys in blogEntries) {
console.log(keys)
}
Functions
… can be typed in TypeScript. The parameters and return values should be defined in the type.
function addStringAndText(figure:number, text:string):string{return toString(figure) text;}
The most common sequence of letters that TypeScript provides you with is this.
. If you want to address a variable outside the curly brackets, place a this.
in front of the variable name:
const PI:number = 3.141;
function calculateCircle(r:number){
return r*r*this.PI
}
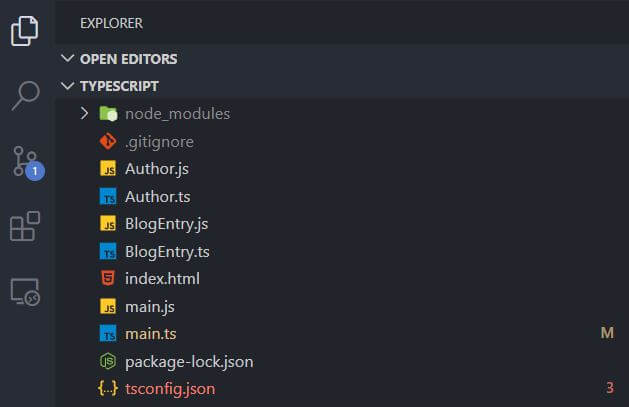
Installation from TypeScript to Hello World
Download Node.js.
Install Node.js with the graphical installer to use NPM in the console.
Execute the command in the CMD / Bashnpm install -g typescript
Create a project folder with a ..
HTML base file index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Typescript Tutorial</title>
<script src="main.js"></script>
</head>
<body>
</body>
</html>
TypeScript file main.ts
import { differenceInYears } from 'date-fns';
import { Author } from './Author';
import { BlogEntry } from './BlogEntry';
var markHartmann = new Author("Mark Hartmann", new Date('December 17, 1995 03:24:00'), "Gartenstraße 4");
console.log(markHartmann.toString());
let yearsOld = differenceInYears(Date.now(), markHartmann.birthday);
console.log("Mark is " + yearsOld + " years old.");
let seriesOfBlogPosts = [];
for (let blogID = 0; blogID < 10; blogID++) {
seriesOfBlogPosts.push(new BlogEntry("Cool Title " + blogID, new Date('December 3, 2095 03:24:00'), "Text " + blogID, markHartmann));
}
seriesOfBlogPosts.forEach(entry => {
console.log(entry.toString());
})
Add a tsconfig.json:
{
"scripts": {
"clean": "rimraf dist",
"start": "npm-run-all clean --parallel watch:build watch:server --print-label",
"watch:build": "tsc --watch",
"watch:server": "nodemon './dist/index.js' --watch './dist'"
}
}
CSS file for the stylesheets
The commandtsc *.ts --watch
monitors all changes in the TypeScript files. As soon as a change is recognised, the TypeScript compiler compiles the file into JavaScript.
Insert the JavaScript file generated by the TypeScript compiler into HTML.<script src="main.js"></script>
Write in the main.tsalert("HelloWorld");
Open the HTML file in your browser. You do not need to use XAMPP.
The console should automatically compile the changes to the JavaScript file after saving main.ts.
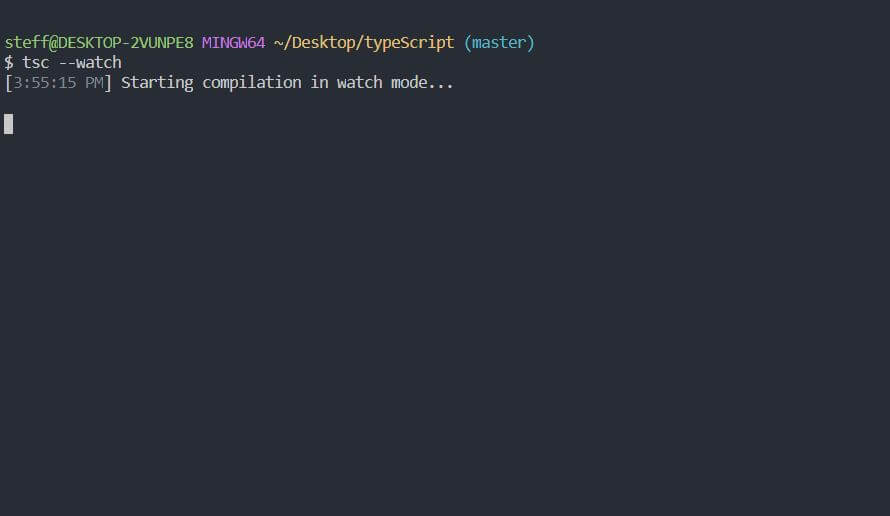
Programming with TypeScript made easy
Deletealert("HelloWorld");
so that we can start a slightly more extensive project.
Create the files Author.ts and BlogEntry.ts to separate the classes from each other
Author.ts
export class Author {
name: string;
birthday: Date;
address: string;
constructor(public nameIn: string, public birthdayIn: Date, public addressIn: string) {
this.name = nameIn;
this.birthday = birthdayIn;
this.address = addressIn;
}
toString(): String {
return "Name: " this.name ", Birthday: " this.birthday ", Address: " this.address;
}
}
BlogEntry.ts
import { Author } from './Author';
export class BlogEntry {
title: string;
publishedAt: Date;
text: String;
author: Author;
constructor(public titleIn: string, public publishedAtIn: Date, public textIn: string, public authorIn: Author) {
this.title = titleIn;
this.publishedAt = publishedAtIn;
this.text = textIn;
this.author = authorIn;
}
toString() {
return "Title: " this.title ", publishedAt: " this.publishedAt ", text" this.text ", author: " this.author.name;
}
}
Save the files.
Reload the browser
Enter in the consolenpm install date-fns
to install a date format package
Enter in the consolenpm install --save typescript nodemon npm-run-all rimraf
to install a cleanup tool for the build processes.
Enter in the consolenpm i nodemon
to view the changes in real time within the console
Create a tsconfig.json where you can enter all compiler settings for the build
Start withtsc -watch
the automatic compilation of the TypeScript files to JavaScript
Start the real-time output of Nodemon with the wordnodemon
INSIDE another terminal so that the TypeScript compiler can continue working undisturbed. All console output should be visible in the terminal window where you started Nodemon. You can check your results there.
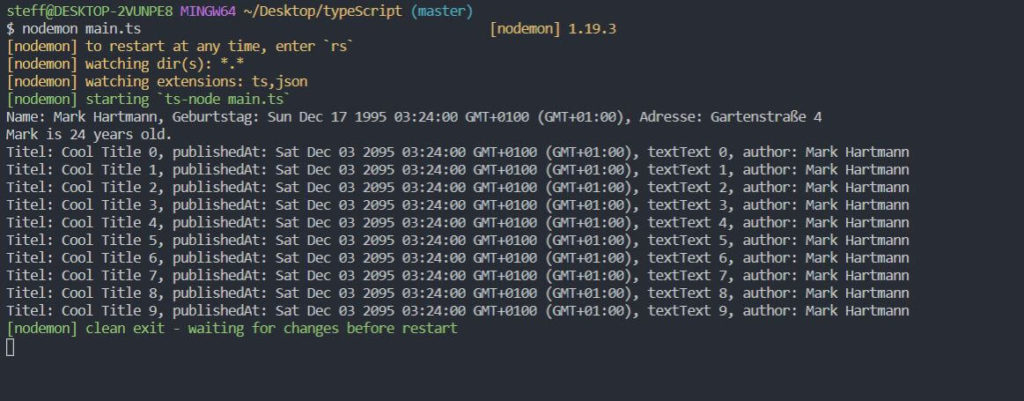
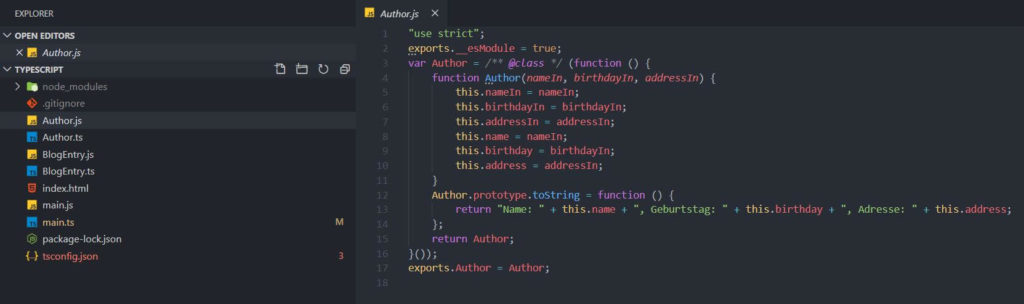
Explanations of the code
The import { X } from 'X';
inserts the code into the main.ts script via a code injection. A class consists of the attributes with the types (optional), followed by the constructor to initialise the class.
Extensions: How can I extend TypeScript?
Haven’t had enough of the TypeScript tutorial?
Then add several Node modules.
Most important NPM commands
To get off to a good start with TypeScript, you should know the most important NPM commands and tricks for using external modules.
Other JavaScript / TypeScript developers have developed modules for time formatting, UI elements, algorithms, etc. in recent years.
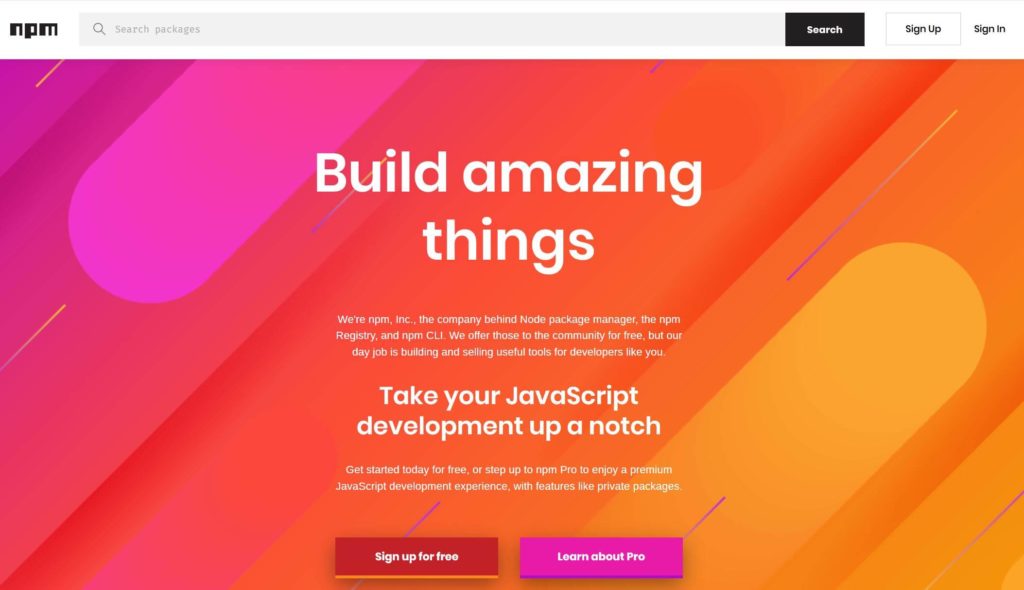
- After checking out of the repo, you can install the Node.js modules because they are not stored in the repo due to memory space:
npm i
- If you need a new module, the command
npm i modulName
installs the module. Via an import statementimport {modulName} from 'modulname';
the TypeScript file can reach the module
- If you want to delete the node_modules folder, use Rimraf
npm install rimraf -g
or for Linuxrm -rf node_modules
Depending on the size of the project, deletion may take longer because Node.js uses a very nested structure. Delete withrimraf node_modules
- In my experience, Node.js “spins” every 3 – 4 days. Simply delete the cache
npm cache clean -force
- You can delete individual modules with
npm uninstall modulName
- The Node.js developers update their modules regularly. You may have to adapt your code due to new versions. Update the modules with
npm update
to eliminate critical errors / bugs / vulnerabilities. - You can install modules globally or locally with Node.js. Modules such as Ionic, Angular or Rimraf install other modules and create their own projects. The -g at the end of the installation command causes the installation to take place within the user folder / AppData.
npm i ionic -g
Conclusion: Your first TypeScript script
TypeScript is very versatile.
Try it out for yourself.
- Write about error messages that occur …
- Criticism with suggestions for improving the tutorial…
- Installation problems with TypeScript …
Thank you. I look forward to your feedback