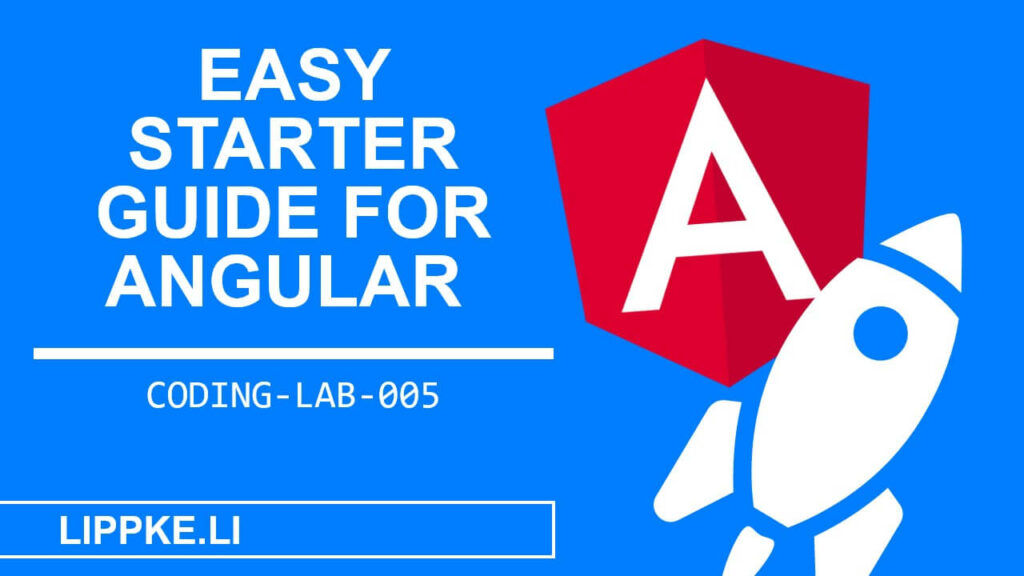
Do you need an Angular tutorial? or …
- Are you planning your own frontend?
- Don‘t want to do a lot of work for yourself?
- Do you want to be able to show a stable UI intelligently with little code?
Since 2016, Angular, a popular web frontend, has been simplifying GUI development.
As for any framework, the developer needs a solid start to learn the concepts and architecture of the framework.
I want to give you that first push with this Angular tutorial.
Let’s get started!
Your first Angular App
We will build a disco Angular app that responds intuitively to your actions.
The tutorial gives you a first introduction to developing an app with the Angular framework for beginners.
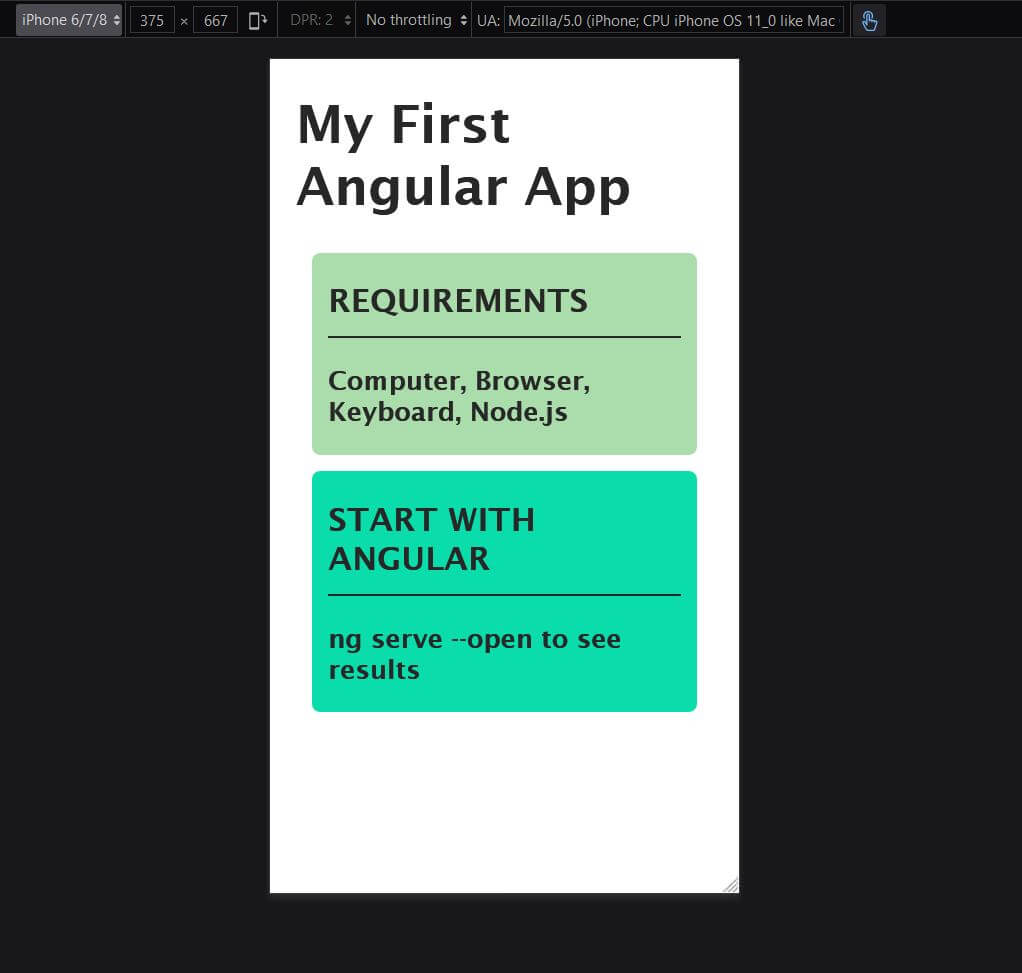
Requirements: Your knowledge
- Have programmed something … with Java, Python or any other programming language
- Knowledge of HTML and / or CSS is advantageous
- Admin rights to install and uninstall programs
Technical requirements
Node.js and an editor are sufficient to get started.
Node.js (LTS) – Package manager for JavaScript libraries
The Angular framework is based on JavaScript. The developer codes in TypeScript. Use Node.js. The package manager works with some JavaScript libraries that you can reuse in your project.
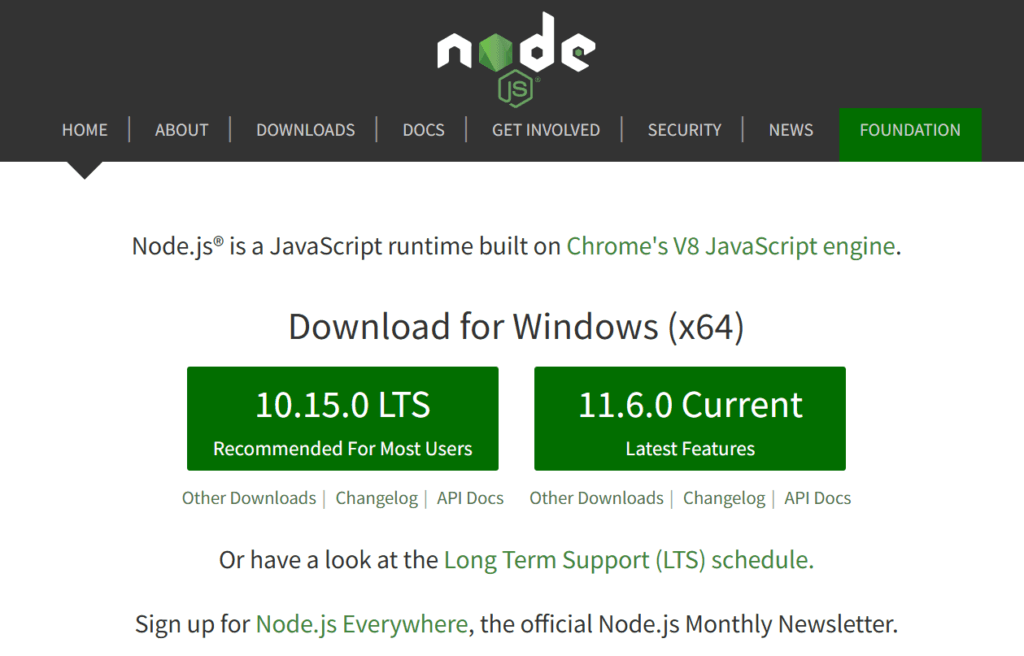
Editor (Visual Studio Code) – Your Digital Canvas
Besides the browser, you need a code editor of your choice. It is best to use an editor with syntax highlighting and IntelliSense such as Visual Studio Code.
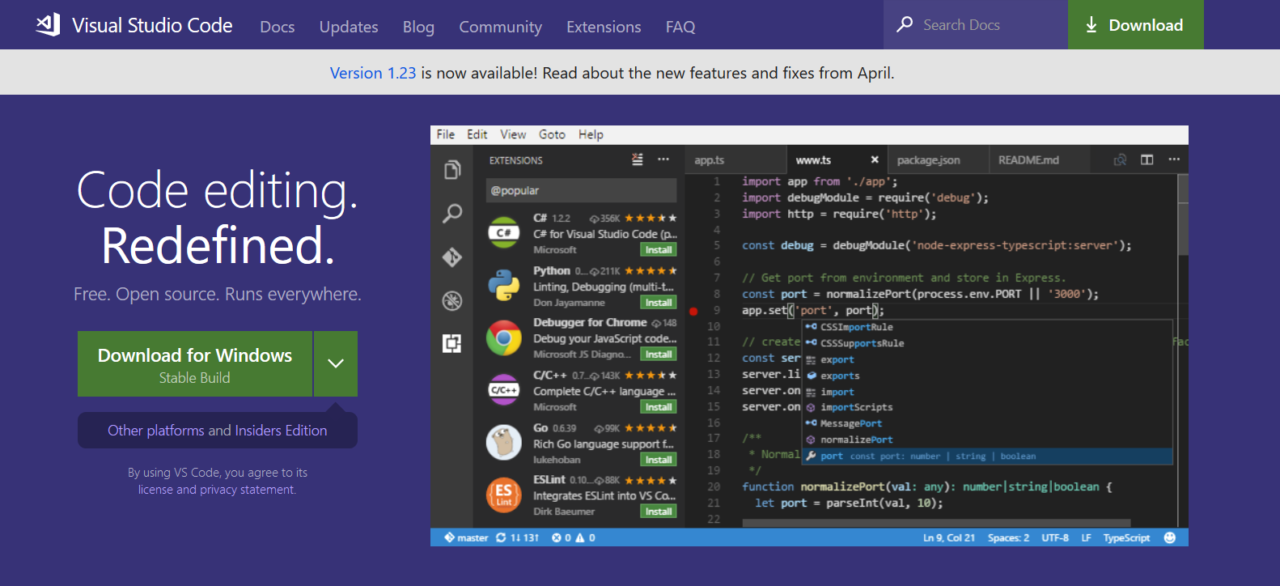
Angular CLI (Latest Version)
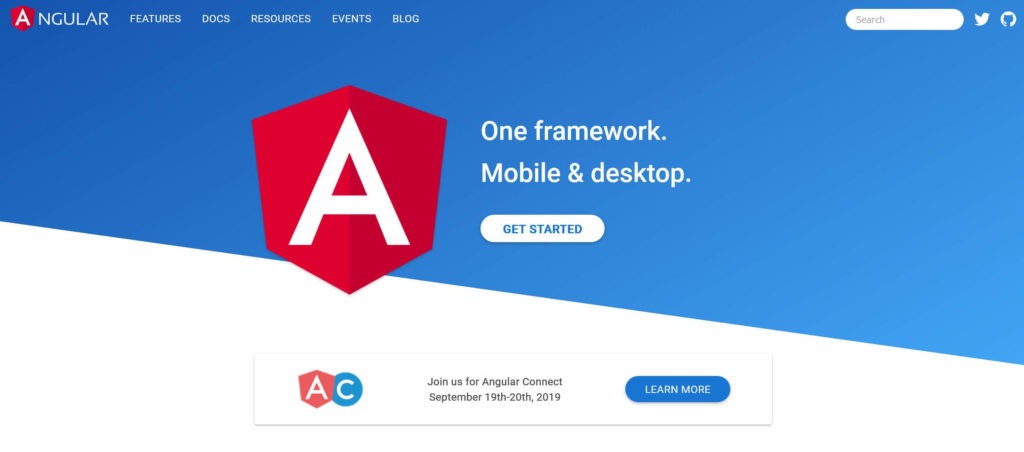
The Angular console (CLI, Command Line Interface) takes a lot of the work out of creating, building and customizing your Angular project.
npm install -g @angular/cli
Concept: Angular basics
A short overview of the most important things about Angular.
I explain the central concepts of Angular.
What is Angular?
Angular belongs to the frontend web frameworks.
The framework is developed by Google.
Anyone can use the open source software (MIT licence) for their own projects free of charge. AngularJS is the predecessor of Angular (without JS). The predecessor is based on different concepts. Syntax and ideas and has changed completely since then.
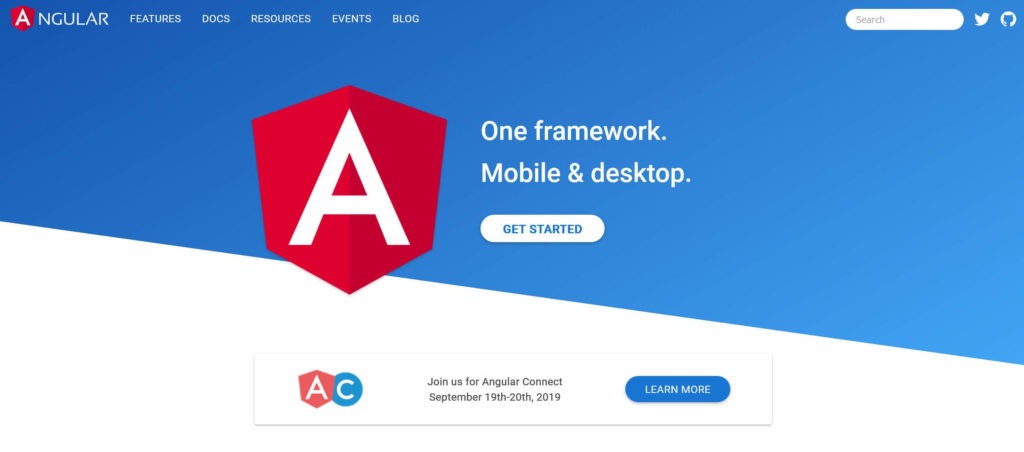
Angular strives for a mobile-first approach, is well suited for desktop applications in the browser in addition to smartphones, and is built on JavaScript Node modules.
The programmer writes Angular in TypeScript, so typed programming is possible.
What is a single-application?
You can develop a single-page web application (SPA) with Angular. SPAs are based on an HTML file, each of which embeds a large JavaScript and CSS file.
The JavaScript provides the content and the application logic. The SPA loads all the pages at the same time, which are stacked on top of each other like stacks of cards. The JavaScript code pushes the requested card to the front.
SPAs are what developers call an offline-friendly application because they don’t have to reload with every interaction.
The framework implements the fat client or rich client so that the programmed application puts less load on the server.
Dependencies Injection – Angular is modular!
Angular = Modular.
The Node.js system consists of JavaScript libraries with reusable JavaScript code.
You don’t need to write a routing module that generates the URL. → User the router
You search for the module on https://www.npmjs.com/, execute the console command npm i modulname
to install modules and import the model.
Since JavaScript 6, the import works with the statement import {Module} from ....
.
The app.module.ts
TypeScript file lists all the modules used as a table of contents. The compiler can more easily convert the TypeScript files into JavaScript code.
The app.module. ts
is the root component that can load all required libraries.
Basics: Angular on your PC
The following section shows you how to get Angular onto your PC.
I explain the important files and directories in an Angular project.
5 steps to get Angular on your PC
- Download Node.js and install the application, the Long Time Support version with the graphical installer.
- Open a PowerShell with admin rights and run
npm i -g @angular/cli
to install Angular on your computer. - Go to a directory where your project should be stored and execute in the shell / bash
ng new myFirstApp
This command creates the Angular project and installs the JavaScript modules. This can take 1 to 15 minutes. During the installation process, the Angular CLI will ask you if you need Angular routing. Angular downloads about 40,000 files from which your app can be compiled. NPM stores the modules inside the node_modules folder, which you can ignore at the beginning. - Type
Y
to confirm. - Select
Sass
as the stylesheet type. - Go into the directory with
cd myFirstApp
- With
ng serve -open
you start the Angular server and your browser shows the app.
Angular’s file and folder structure explained
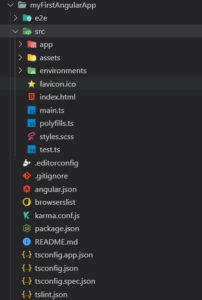
- dist – contains all finished web builds in pure JavaScript.
You program in TypeScript (a related scripting language), which the compiler translates when building the Angular project. - nodes_modules – is where all the JavaScript libraries of Node.js are located.
- src – is the most important folder for development. It contains your TypeScript code. All images and CSS files are located there.
- app – this folder contains the essential code for your app.
- The file extensions stand for…
- .ts are TypeScript files (logic)
- .css or .scss are stylesheets (appearance)
- .html is the basic framework (elements, texts, images)
- .json are the data bases (content, texts in pure form)
- app-routing.module.ts – makes sure that you can navigate to the individual SPA views with a URL, even though there is only one HTML file.
- modules.ts imports the JavaScript modules to make the app work.
- assets – can contain images, icons and fonts
- index.html – is the central HTML file where everything runs together. This imports the compiled JavaScript to the single app.
- css – is the global stylesheet for the whole project
Coding: Off to practice
I hope you were able to install npm and Angular to get a quick and good start with Angular.
Let’s write the first lines of code for Angular now.
This file is the basic framework of the app.
The HTML components specify the content such as texts, images and videos and its structure and arrangement. The box structure is indented with tabs.
<html>
<head>
<title>{{webpageTitle}}</title>
</head>
<body>
<h1>{{webpageTitle}}</h1>
<div *ngFor="let card of cards" (click)="changeColor()">
<div class="card" [style.background-color]="card.colour">
<div class="titleBox">
<h2>{{card.cardTitle}}</h2>
</div>
<div class="content">
<p>{card.cardContent}}</p>
</div>
</div>
</div>
</body>
</html>
A special feature of Angular are the double curly brackets {{}}
. These mark an Angular variable that you can use as ..
- Strings (texts)
{{myText}}
- Number
{{myNumber}}
- Arithmetic operations
{{5 5*5}}
- Formatted data
{{date | date german}}
- Function calls
{{goBack()}
}
…can be used.
Another essential feature of Angular are its own directives. Directives describe the behaviour and appearance of a tag closer e.g. [style.border]=""
.
These “adjectives” describe an HTML tag in more detail.
*ngFor
belongs to the structuring directives. Angular uses *ngFor
as a control structure. Like a For loop, *ngFor
copies a DIV box several times, for example. This is similar to a For loop as in any other programming language.
*ngFor
iterates (repeats) over the array cards
.
card
is an object from the arraycards
tilteCard
accesses a string within a JSON
Angular can access the components of a JSON inside the double curly braces.
src > app > app.component.scss
As explained in my SCSS guide, there are three SCSS variables defined in this file.
$primary-color: #abdcab;
$border-color: #272727;
$normal-margin: 10px;

$primary-color: #abdcab;
$border-color: #272727;
$normal-margin: 10px;
* {
colour: #272727;
font-family: 'Lucida Sans', 'Lucida Sans Regular', 'Lucida Grande', 'Lucida Sans Unicode', Geneva, Verdana, sans-serif
}
.card {
background-color: $primary-color;
border-radius: 5px;
padding: 1px $normal-margin;
margin: $normal-margin;
font-family: Arial;
}
.card h2 {
border-bottom: 0.5vw solid $border-color;
width: 100%;
text-transform: uppercase;
padding-bottom: $normal-margin;
font-size: 20px;
}
A Sass compiler converts the SCSS code into normal CSS code. If you use variables and other functions of SCSS, you can save a lot of time.
The star selection in the CSS refers to all HTML components defined on this web page.
In the CSS classes we use the previously defined CSS variables for the colours and spacing:
src > app > app.component.ts
import { Component } from '@angular/core';
import { default as cardsContent } from './cardsContent.json';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
webpageTitle = 'My First Angular App';
cards;
constructor() {
this.cards = cardsContent.cards;
console.log(this.cards);
}
changeColor() {
this.cards[0].color = "#" (Math.random() * 255 * 255 * 255).toString(16).substr(0, 6);
this.cards[1].colour = "#" (Math.random() * 255 * 255 * 255).toString(16).substr(0, 6);
}
}
The code breaks down into three blocks:
- Import statements
- All dependencies / JavaScript library import these lines of code.
- I imported a locally-hosted JSON file with the contents of the maps.
- Components
- “Bracket”: this is where all the parts that make up a module in Angular come together e.g. the HTML, CSS and JavaScript.
- Export class contains the functions of the app logic.
- First you define the variable
title
andcards
(2D array) - The constructor loads the content into the
cards variable
. - The function
changeColors()
:- Lines 21 and 22 are to overwrite one of the JavaScript arrays with a new RGB code.
- The
Math.random()
function generates a number between 1 and 0 with many decimal places when executed. - The standard RGB colour palette provides you with a spectrum of
255
shades of red combined with255
shades of green and255
shades of blue. .toStirng()
function converts the decimal number to hexadecimal, as is usual for RGB code..substr()
removes all numbers after the decimal point. An RGB code is usually a 6-digit hexadecimal code (with transparency levels 8 digits).
- First you define the variable
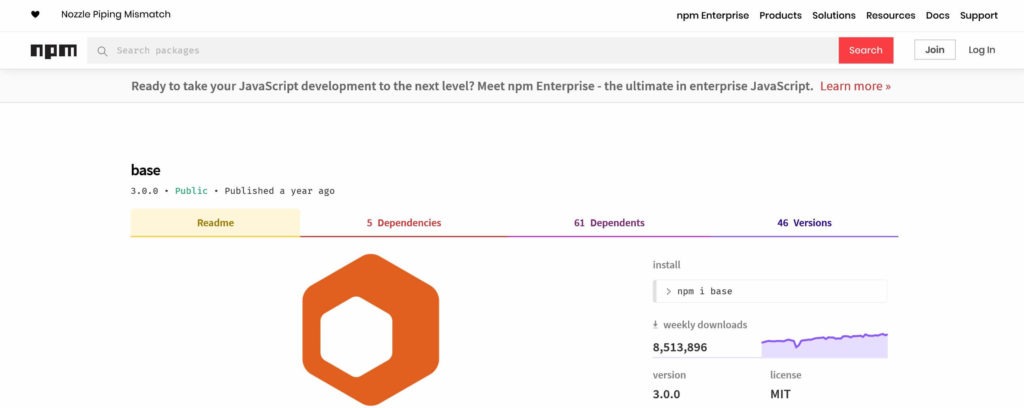
src > app > cardsContent.json
{
"cards": [{
"cardTitle": "Requirements",
"cardContent": "Computer, Browser, Keyboard, Node.js",
"color": "#abdcab"
}, {
"cardTitle": "Start with Angular",
"cardContent": "ng serve --open to see results",
"color": "#0bdcab"
}]
}
The JSON stores the strings for the cards.
Later we can move the JSONs to a NoSQL like MongoDB or CouchDB which simplifies the data storage.
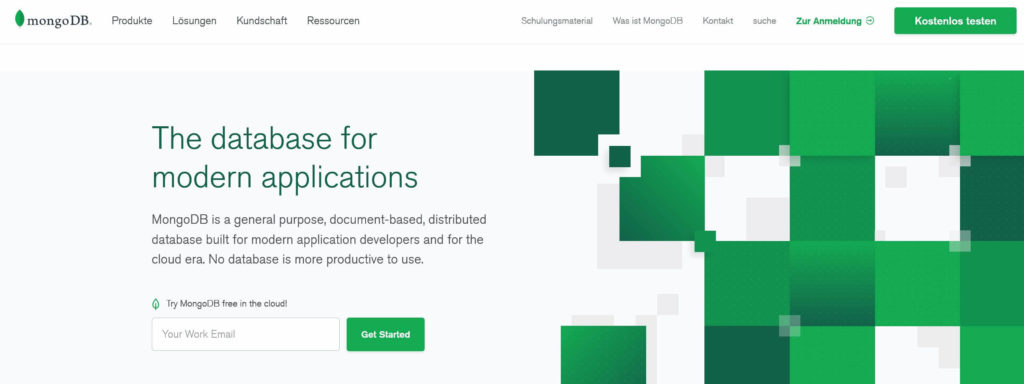
The cards each contain a title, text content and its initial colour.
Extensions: How can I extend the app?
Haven’t you had enough of the Angular tutorial yet?
Or would you like to learn more about Angular?
Then I’ll show you in this section how you can extend your app and your knowledge.
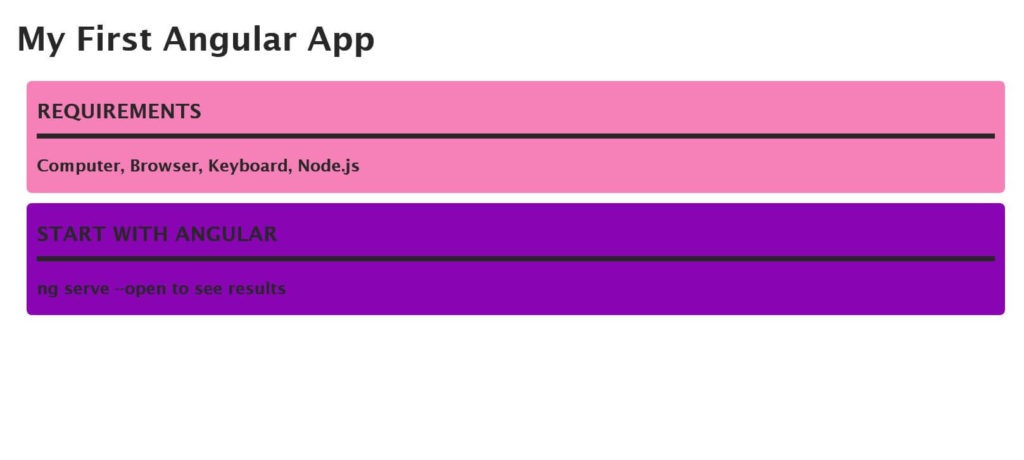
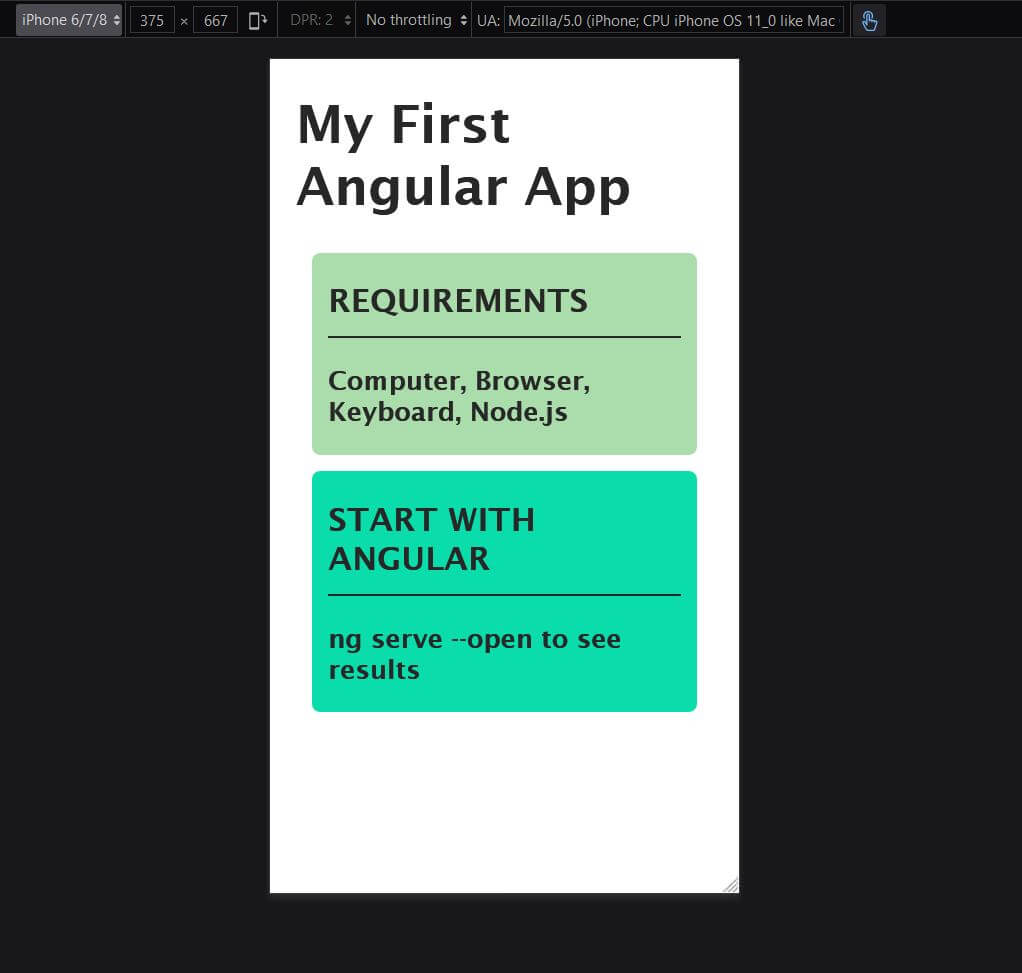
- Add more cards
- Change the styles to your liking
- Fade in and out cards with *ngIf
- Add a CSS animation e.g. a transition
- Try ng-Bind
Angular 12? Which is better?
Angular 12 bring cool new features:
- Form are easier to use (@angular/forms)
- Smaller file end size after build due to a Zone.js opt-out Optional dependencies
- Testing is much faster
- Code splitting
- Optional dependencies and modules
Angular 9 brings some changes with Project Ivy since 7 February 2020:
- Angular apps are smaller after building: Ivy can reduce file sizes to up to 40% (for large apps). This means that the Angular app loads faster.
- Testing with Angular is faster
- New debugging features with nicely readable stack traces: you can now easily trace your errors
- Improved connection between CSS and the HTML framework
- Fewer build errors: even if a build error happens, you can more easily trace the error back to the basics.
- Build time reduced by developers. Internationalise your app with the i18n replacement for different languages.
- Test out the new YouTube player
- … or the new Google Maps component can beautify your new Angular App
Update and enjoy
Users of Visual Studio Code can look forward to the language service improvements that make typing Angular lines even easier.
The new features don’t affect beginners for now. Learn to work with the latest Angular version.
To use Angular 12 in your project, it is recommended to transfer module by module.
Conclusion: Your own Angular app
Be creative. Read the documentation. Test Angular.
Angular is versatile and (after a period of getting used to it) lean, flexible and useful. Don’t miss the chance to build new web applications with Angular.
When you are done with an Angular project, you can create an installable version with ng build -prod
. Upload this folder to a (normal Apache) web server.
I am looking forward to your comments with the link to your (first) Angular project.
- Write about errors that occur …
- Text passages that were unclearly described
- Criticism with suggestions for improvement…
- Suggestions for new posts …
- Installation problems …
Thank you. I look forward to your feedback