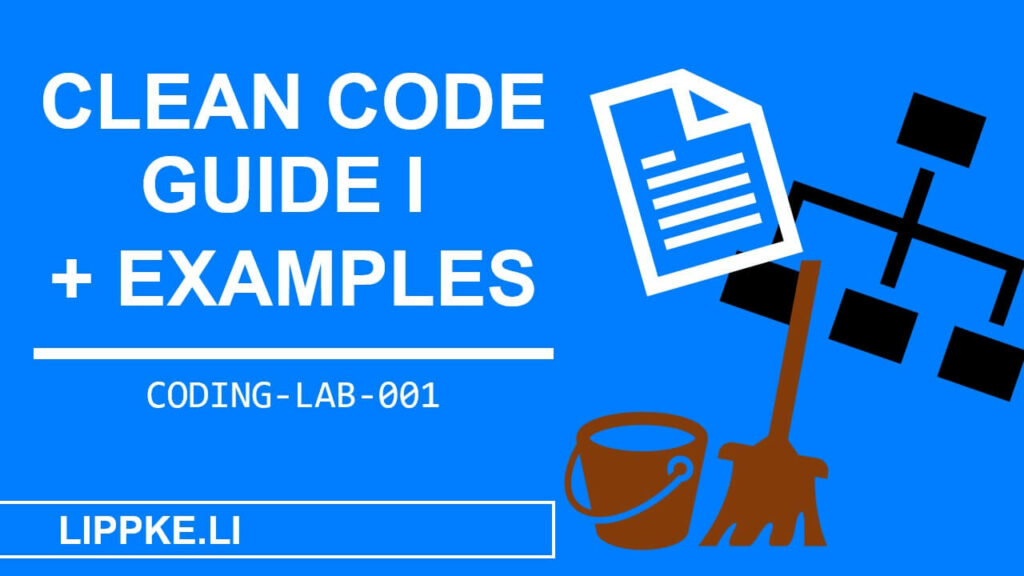
Want to write clean code?
This 3-part tutorial gives you the code examples with tips and tricks for practice!
Let’s get started!
What is clean code?
Clean code consists of logically structured and intuitively named variables and functions that anyone with a basic knowledge of the programming language can overview and understand in a reasonable amount of time.
6 reasons not to use clean code
For most beginners, clean code sounds like fairy writing at school: useless!
But what happens when you use dirty code? Here are the problems:
1. Dirty code is expensive
The development and maintenance of larger coding projects with dirty code takes longer and is therefore more expensive. With dirty code, collaboration is more difficult, and you lose the overview more quickly.
2. Faster to bankruptcy
Some software companies have gone out of business because they couldn’t fix their software bugs in time. The code was so confusing that debugging was very time-consuming. Support contracts expired and customers no longer wanted the products. With exploits, software manufacturers have to deliver a patch in a matter of days to hours.
3. Follow the Employeer Rules
Some employers have strict naming conventions and regulations that you must adhere to as a programmer. If you don’t follow the rules, you will end up in first-level support.
4. Making your work more difficult
Every programmer knows it. With a large document (500 lines or more) and bad code, you can lose track of the functions and variables. Every additional feature makes working with dependencies much more difficult.
5. Nobody wants to read or maintain your code
If a user finds a bug in your programme, then the software manufacturer is obliged to make improvements. You are not always the person who has to solve the bug, but another developer.
6. Demands on you
Even if you are not a professional developer, you should take care of your code. An author should not rename things on a whim.
Remember: some developers take clean code too seriously and take coding to extremes. Your clean code doesn’t have to be 100% perfect, but should serve the purpose of helping others and yourself understand the code.
That’s why I’ve written a 3-part guide with lots of examples to show you what clean code can look like.
The optimum
To illustrate what clean code is, I would like to give a comparable example:
An author like King writes books that are so well written that the reader can read them in one flow (given enough time). Good code should be like a good book. Your programming code is the fluent explanatory text for the programmer, which a computer can also understand.
What does clean code have to do with English sentences?
A English sentence essentially consists of nouns, verbs and adjectives.
In the context of programming, …
- Nouns are classes, variables and objects (var order, seller, amount)
- Verbs are functions (getOrders(), setName())
- Adjectives are parameters (startTime, endtime)
From English language to clean code
Example: You want to convert minutes into hours.
In English, you explain it like this:
- Calculate the number of hours by dividing the number of minutes by 60, rounding down and returning the number of hours.
- Calculate the number of minutes by taking the number of minutes modulo 60 and returning the value.
var minutenAnzahl = 129;
calculateNumberOfHours(){
var hours = minutenAnzahl / 60;
return Math.floor(hours);
}
calculateNumberOfMinutes {
return (minutenAnzahl % 60)
}
In computer science, an algorithm describes a defined sequence of steps. You will be familiar with “analogue” algorithms from cookery books or the assembly instructions for your cupboard.
Motivation – Why should I use clean code?
Why should I read the guide?
Let’s start with the code style practised by many professors and lecturers of distinction at many universities:
var x1 = 9;
var y2 = 3;
function kgt(x1, y2){
for(let i = 0; i <x1; i++){
if((x1 % i) == 0 && (y2 % i) == 0 ){
return x1
}
}
}
As the code is small, you can assign the variables. However, if 100 variables and functions only consist of cryptic abbreviations, you will quickly lose track of the code.
Variables and naming in clean code
A good variable / function has 8 – 24 characters, because a variable that is too short is not clear enough and one that is too long is difficult to read.
Some companies prescribe the naming schemes for programmers, and you must adhere strictly to them. If you are programming privately, you should observe the following rules:
Name your variables, functions and parameters with names that accurately describe the exact content or task.
Take time for the naming and find a suitable name.
The naming rules apply to file names, fonts, folders… or anything you can name in a software project.
Change a variable if you have found a better name.
👌 Clean code – this is what your code should look like
Clear, concrete. Understandable. The name is the content of the data structure. Be as specific as possible with the name.
var hourOfOrder:number;
String articleDescriptionJSON;
var checkoutPaymentSum: number;
getSurNameClient()
changeOrderStatus()
sumProductsOfOrder();
Functions in clean code
Functions are the verbs of good code. A function name describes the action that the function fulfils. Prefixes such as get, set, load, update, parse, …. are typical for function names
Name functions with similar tasks with the same prefix!
Fetch- and get- mean the same thing, but remain consistent in naming. Sorting and bundling similar functions is easier with the same prefixes.
The shorter a function, the better. My recommendation is 5 – 70 lines.
Functions should be short because a function should only do one thing:
- Execute HTTP GET
- Parse HTTP content
- Error handling
A short function has the advantage that you have a complete overview and can divide the code into small sections that you can reuse (DRY principle).
Cascade your functions!
If you have a lot of code, then you need to cascade your functions.
Imagine a tree. The root of the tree is the main function that the computer executes first. Then you call the sub-functions. The sub-functions may use other sub-functions.
💣Coding horror – Not like this!
Please do not use general terms. Be specific. Avoid long functions and don’t pack everything into one function!
function functionAll(){
doSomeCrazyStuffNobodyUnderstands();
machineLearningTurnOn();
[... 400 lines of code]
callHelperOfHelperClass();
doSomeCrazyStuffNobodyUnderstands2();
}
👌 Clean code – this is what your code should look like
Create abstracted levels of function cascades. 1 function = 1 task and no more, please.
main() {
loadOrdersInMemory();
prepareOrdersToDisplay();
displayOnGUI()
}
loadOrdersInMemory(){
getOrdersByHTTP();
getUserProfile();
}
prepareOrdersToDisplay() ...
Classes as clean code
The classes are the nouns of our clean code.
Your classes should describe people, things and concepts. The attributes of a class are the adjectives. All names should be chosen carefully.
Stick to standards such as
z. For example, each class should contain the typical CRUD (create, read, update, delete) methods.
I recommend a toString() method for each class, which should quickly provide information about the content of an object of a class during debugging. In Spring, the annotation @ToString is sufficient.
Model real concepts and observe standards in classes
💣Coding horror – not like this!
The classes describe concrete concepts and objects… and not “information”
class OneThing(){
x1;
contructor(x1){
this.x1 =x1;
}
infoFunction(){
return x1;
}
}
👌 Clean code – this is what your code should look like
Represent reality. With terms that everyone is familiar with.
class Order(){
isSent:boolean;
constructor(isSent:boolean){
this.isSent = isSent;
}
getSendStatus()...
setSendStatus()...
}
Learnings for this chapter
- Good code is a good user manual
- Clean code saves time and money
- Name your variables, functions and parameters with names that accurately describe the exact content and task.
- Name functions with similar tasks with the same prefix
- Cascade your functions
- Model real concepts and observe standards in classes
Next chapter
Chapter 2: Clear structures and format for clean code
The outer framework of code is used to maintain an overview of the project and complete projects more quickly.
Chapter 3: Clean code is better: shortening and optimising
Clean code is not just a shell. Really good code is easy on memory and CPU!