
Want to learn JavaScript?
Get into the JavaScript universe with this guide, with which you can develop feeds, apps, games and more!
By the end of the tutorial you will have programmed your first JavaScript app!
Let’s get started!
Why should I learn JavaScript?
The internet is growing and growing. JavaScript is the heart of the internet.
Steffen Lippke
Websites that used to be only showed static content (text and images) are transformed into interactive applications with the use of JavaScript.
JavaScript not only drives business and productivity software, but many browser games and apps are based on JavaScript.
JavaScript is the basis for a whole programming universe: Node.js and all JavaScript frameworks.
A web page with JavaScript can change and process user data without reloading. The browser can reload new content via the Ajax function.
JavaScript is not only a programming language for client-side web page programming, but works with Node.js on the server.
What is JavaScript?
The JavaScript programming language enables interactive and dynamic web pages. Programmers can use this programming language to fill the static content of the website (HTML) with life. The user can interact with the website while the website does not have to reload.

The programming language JavaScript is popular because every JavaScript application runs on (almost) all operating systems that support a modern browser such as Chrome, Firefox or Opera support.
The more advanced JavaScript technologies Accelerated Mobile Pages (AMP) and Progressive Web Application (PWA) remove the boundaries between applications, apps and websites.
More efficient programming – frameworks
Most developers rarely use JavaScript in a pure form. They either use libraries to avoid duplicate code or frameworks.
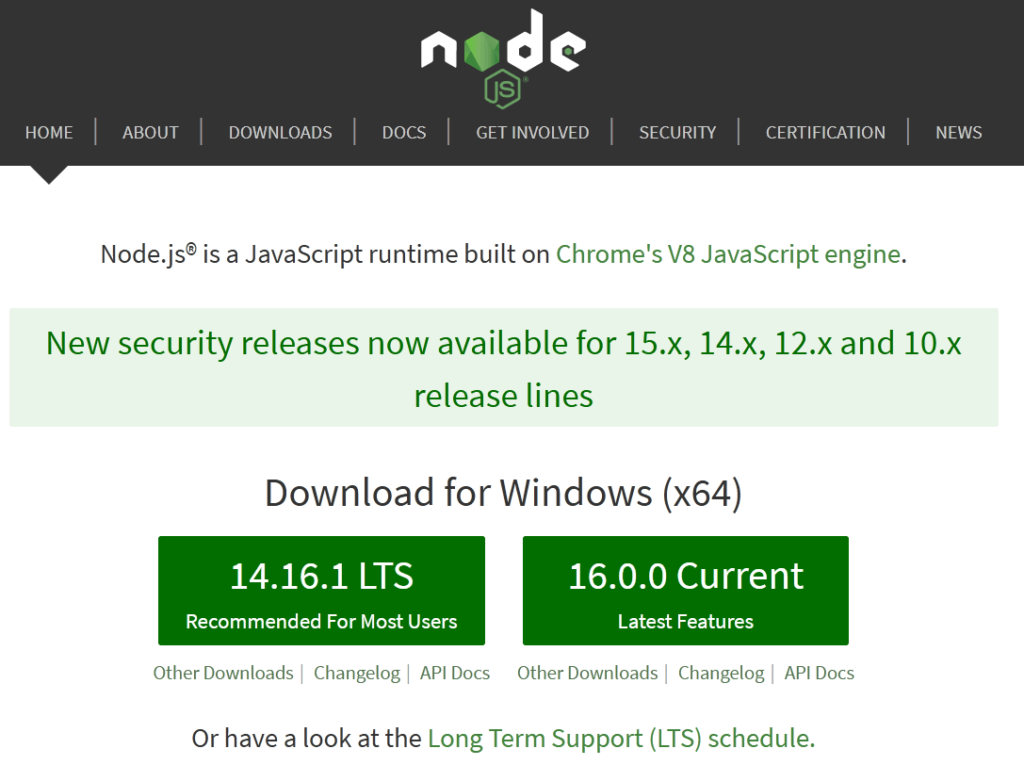
JavaScript frameworks are e.g. Vue, Angular, Ionic.
Many frameworks used the further development TypeScript instead of JavaScript.
Steffen Lippke
TypeScript improves JavaScript programming by structuring and typifying JavaScript. Many developers love the typing of variables, as well as a clearly arranged dependency management (unlike JavaScript).
The TypeScript compiler translates the TypeScript code into JavaScript code, which the browser interprets.
Beginner Tutorial JavaScript
Installing the development environment
As a development environment I recommend the free Visual Studio Code. Unlike many other development environments, it is not overloaded and can be customized with extensions.

Your first Hello World
Create the following HTML document. If you want to know more about HTML, read this guide.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
</body>
</html>
In the head of the HTML document, add a <script>
tag that contains the JavaScript code. You can call up the HTML file via the browser.
If the JavaScript code is inside these brackets, the browser executes the code when the web page is loaded.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script type="text/javascript">
/* Hier kommt JavaScript herein */
</script>
</head>
<body>
</body>
</html>
You can use a JavaScript event to trigger a function. An event is, for example, the click event (on a link / button) or the “loaded event” when the browser has completely displayed the web page.
Complete your Hello-World in JavaScript with a dialogue (alert):
<script type="text/javascript">
alert("Hello World!");
</script>
Your new calculator
We need two input fields (input), a dropdown menu (select) and a button (button) for a calculator. The user should enter the numbers into the 2 input fields and select the operand with the dropdown in the middle.
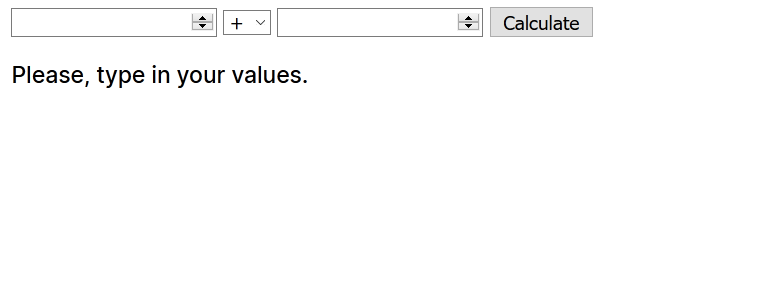
At the end, we give the result in an output text.
The function calculate()
encapsulates the JavaScript code that called the onClick
attribute.
We get the number inputs by referencing the HTML objects in the JavaScript code by name (document.getElementByName("…")
).
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<script type="text/javascript">
function calculate(){
var operand1 = parseInt(document.getElementById("operand1").value);
var operand2 = parseInt(document.getElementById("operand2").value);
var result = 0;
switch (document.getElementById("operator").value) {
case "plus":
result = operand1 + operand2;
break;
case "minus":
result = operand1 - operand2;
break;
case "devide":
result = operand1 / operand2;
break;
case "multiply":
result = operand1 * operand2
break;
default:
result = operand1 + operand2;
break;
}
document.getElementById("result").innerHTML = "Das Ergebnis ist: " + result;
};
</script>
</head>
<body>
<input type="number" id="operand1" />
<select id="operator">
<option value="plus">+</option>
<option value="minus">-</option>
<option value="devide">/</option>
<option value="multiply">*</option>
</select>
<input type="number" id="operand2" />
<button onclick="return calculate();">Calculate</button><br />
<p id="result">Please, type in your values.</p>
</body>
</html>
We store the values of the contents in a variable marked with var
. JavaScript does not use strict typing. You do not have to specify which variable type you use. You can overwrite the operands with text, JSON and Boolean.
The JavaScript programming language does not care about overwriting with other types.
A switch
statement is necessary to convert a string into a mathematical operation. The operations take place within the switch
statement.
Finally, we write the result in the output text via the innerHTML
-Function.
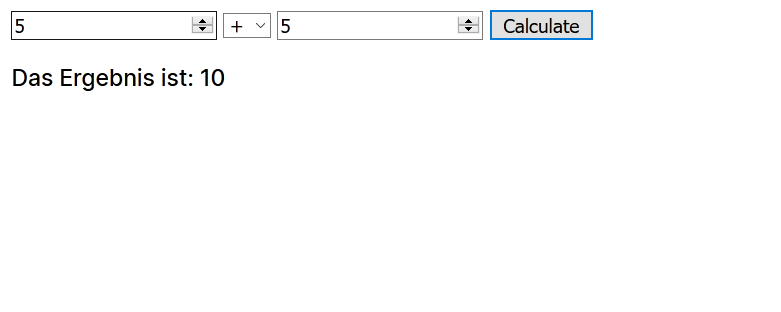
Load content afterwards – Ajax
JavaScript is suitable for reloading content after the initial page load.
The website can use this technology to display images with a delay in order to display the text more quickly. Ajax loads new blog posts of a website without the browser having to perform a new page load.
Libraries and Node.js
The Node Package Manager (NPM) provides JavaScript developers with a variety of libraries that can speed up development. The universe of Node.js offers everything from small helper scripts to full frameworks with all the features for a business application.

You can load the libraries via the import
statement.
Before you start with Node.js, I recommend you to read the TypeScript Tutorial to read.
How do hackers use JavaScript?
JavaScript not only offers benefits to users, but can also act as a malware. Hackers use JavaScript to attack their victims:
- Overlay login: a JavaScript script overlays a login mask and uses this deception to grab customers’ passwords from.
- Crypto-Miner: A web page or an embedded advertisement lets your browser Code execute that mines Bitcoins for the hackers in the Hidden mines. The victims get to pay the electricity costs for mining, while the hackers make good money from the Crypots.
- Attack vector: JavaScript can trigger a fully automated malware download trigger, which Your Which can infect your computer. You don’t even have to click on a download link for the malware to land on your computer.
- Integrations: The hackers can spy on you using the browser’s camera and microphone integration.
You can effectively protect yourself from most of the above hacks with these tips. You should be aware that many websites work like normal desktop applications.
When is JavaScript a good choice?
Write an application with JavaScript if the software …
- … should be available on every platform … Windows, macOS and Linux.
- .. can run in a browser.
- … the client has sufficient computing power for the applications.
Alternatives for JavaScript
One of the best alternatives to JavaScript is WebAssembly.
This allows the execution of C++, Rust and C on Your Computer via the browser. The language creates the possibility that computationally intensive applications like…
- Graphics editing software
- Video editing software
- Games
- CAD software
- …
… no longer need to be installed. The applications are quickly available in the browser.
If you can code C(++) or Rust with C(++) or Rustyou can start with WebAssembly. JavaScript is well suited for many programming novices because the programming language “forgives errors”. The software does not crash immediately, but executes the functions that work.